This post is about how to view the world with advection-equation glasses for simulating computational fluid mechanics. I will be discussing how to derive the numerical flux of for a coupled set of linear (purely hyperbolic) partial differential equations by decomposing them into propagating waves, and how to implement a simple finite volume scheme.
Helloworld922's Blog
A blog about whatever seems to interest me.
Tuesday, March 17, 2015
Tuesday, November 25, 2014
Experimenting with Diodes and Non-Linear Curve Fitting
How much does a diode's characteristics actually diviate from diode to diode? I measured a small number of diodes, and then recovered the SPICE DC parameters. Due to the limitations of my equipment, I only did this in the DC forward biased region. I'll also describe how to accurately recover the SPICE parameters using numerical methods.
Tuesday, April 15, 2014
Don't Throw Away your Common Sense
Just because you have a fancy computer machine, don't throw away your common sense. To find out why, just click this link: \(\sin(2 \pi x) = 0\)
If Wolfram fixes this, here's a screenshot to savor this moment:
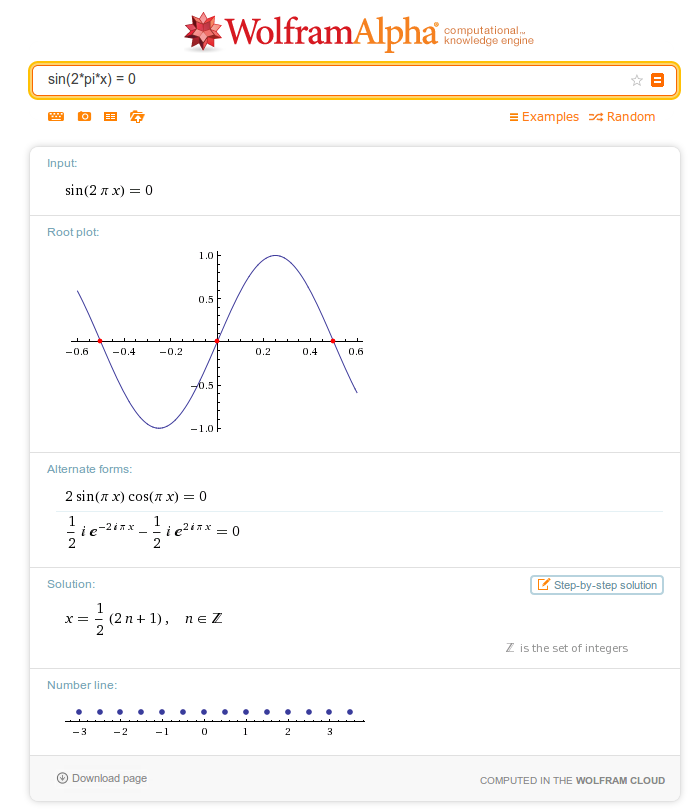
If you don't know what's wrong, well perhaps you have already thrown away your common sense...
Tuesday, August 6, 2013
Signals3 Source Code and Performance Testing Update
Introduction
Since my last post on a fast thread-safe Signals/Slots implementation I've had to do a major re-write. The problem was that I had merged "slots" with nodes. This made implementing "self-disconnecting" slots near impossible (or at least, very inefficient). I also had structured my code quite poorly so managing includes was a nightmare.
Tuesday, July 30, 2013
Signals/Slots Improvements: Better and Faster
Introduction
Last time I presented basic work on my implementation of Signals/Slots which was thread safe, full-featured (well, is capable of being full-featured), and performed quite well. I had a decent implementation, but there were problems with passing iterators. I have figured out a better solution by making a small change. This improves performance, as well as easily allows the use of begin/end iterators rather than a single iterator which tracked all state.
Tuesday, July 23, 2013
Thread-Safe Signals/Slots using C++11
Introduction
For any C++ developer who's used Qt, we've grown to love the Signals/Slots idiom it presents for creating clean Observer code. However, it relied on the Qt Moc pre-compiler tool, which meant any project that wanted to use this feature had to use follow along with the Qt idiom, which really made Qt applications look potentially foreign despite being written in C++. In addition to this the implementation wasn't type-safe (a.k.a. no compile-time type checking), and doesn't generalize to any callable target (you have to extend QtObject and declare a slot using Qt's syntax, can only return void).
Since then, there have been multiple implementations of Signals/Slots. Some notable ones are listed below:
- Boost Signals. Not thread safe, performance wasn't great, now deprecated in favor of Boost Signals2. Licensed under the Boost Liscense.
- Boost Signals2. Thread safe upgrade of Boost Signals. Others have complained about its performance, but my tests seem to show it's at least decent.. Licensed under the Boost Liscense.
- Libsigc++. Supposedly decently quick, but not thread safe. I think this is also a near-fully featured implementation like Boost Signals/Signals2. Also licensed under LGPL, making use somewhat restricted.
- libtscb. A thread safe fairly quick quick implementation. However, it skimps on features (I think it offers similar features to Qt's implementation). Also licensed under LGPL.
- C++11-based Implementation. This is actually another blog which sought to implement Signals/Slots using new features brought by C++11, namely variadic templates, std::function, and possibly more. This is CC0 licensed (public domain). Probably one of the fastest implementations I have seen, but is not thread-safe.
I was wondering if I could implement a more feature-full implementation like Boost Signals2 bet with better performance like libtscb. I'll be using some C++11 features like the last implementation, notably atomics, std::function, and variadic templates. I'm also using Boost shared_ptr/weak_ptr, Boost mutex, and Boost shared_ptr atomics. These libraries are being used because currently the compiler I'm using doesn't have the standard variants implemented (Mingw w64 gcc-4.8.1).
For the most part, I was able to implement (or actually, can see a straight-forward implementation) nearly all of the features provided by Boost Signals2. There are some semantic changes (notably the interface for combiners is different), and I was unable to capture the O(log(n)) performance of inserting group-ordered slots. My implementation likely will have O(n) group insertion.
Tuesday, June 25, 2013
Wheel Factorization with Variable Increments
Just a quick update to my previous wheel factorization code. I found a better way to implement the wheel factorization code which doesn't use bit masks to sieve out values. This version has a decent performance boost, and I think is easier to implement.